API reference¶
safir Module¶
safir.arq Package¶
An arq client with a mock for testing.
Functions¶
|
Construct Redis settings for arq. |
Classes¶
|
A base class for errors related to arq jobs. |
|
Mode configuration for the Arq queue. |
|
A common interface for working with an arq queue that can be implemented either with a real Redis backend, or an in-memory repository for testing. |
|
Information about a queued job. |
|
A job cannot be found. |
|
The job was not successfully queued. |
|
The full result of a job, as well as its metadata. |
|
The job's result is unavailable. |
|
A mocked queue for testing API services. |
|
A distributed queue, based on arq and Redis. |
|
Configuration class for an arq worker. |
Class Inheritance Diagram¶
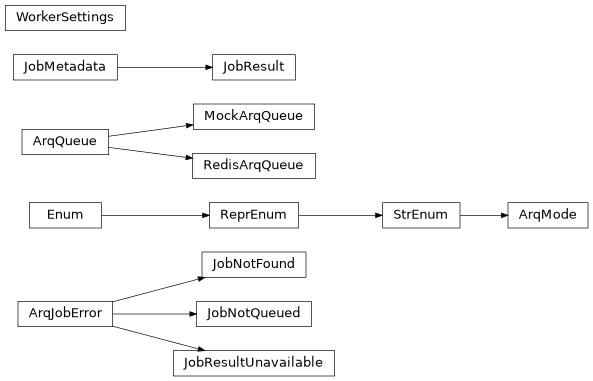
safir.arq.uws Module¶
Construction of UWS backend workers.
Functions¶
|
Construct an arq worker for the provided backend function. |
Classes¶
|
Minimal configuration needed for building a UWS backend worker. |
|
An error occurred during background task processing. |
|
Types of errors that may be reported by a worker. |
|
Fatal error occurred during worker processing. |
|
Metadata about the job that may be useful to the backend. |
A single result from the job. |
|
|
Transient error occurred during worker processing. |
|
Transient error occurred during worker processing. |
|
Parameters sent by the user were invalid. |
Variables¶
Name of the arq queue for internal UWS messages. |
Class Inheritance Diagram¶
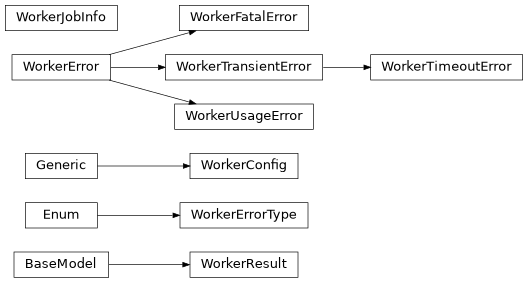
safir.asyncio Package¶
Utility functions for asyncio code.
Functions¶
Run the decorated function with |
Classes¶
An asyncio multiple reader, multiple writer queue. |
|
Invalid sequence of calls when writing to |
Class Inheritance Diagram¶
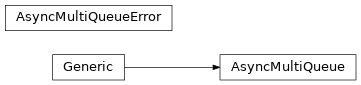
safir.click Package¶
Click command-line interface support.
Functions¶
|
Show help for a Click command. |
safir.database Package¶
Utility functions for database management.
Functions¶
|
Create a new async database session. |
|
Create a new async database engine. |
|
Add the UTC time zone to a naive datetime from the database. |
|
Strip time zone for storing a datetime in the database. |
|
Drop all tables from the database. |
|
Create and initialize a new database. |
|
Check whether the database schema is at the current version. |
|
Retry if a transaction failed. |
|
Run Alembic migrations in offline mode. |
|
Run Alembic migrations online using an async backend. |
|
Mark the database as updated to the head of the given Alembic config. |
|
Mark the database as updated to the head of the given Alembic config. |
|
Clear the Alembic version from the database. |
Classes¶
The Alembic configuration was missing or invalid. |
|
|
Paginated SQL results with pagination metadata and total count. |
|
Run database queries that return paginated results with counts. |
Database initialization failed. |
|
|
Pagination cursor using a |
|
The provided cursor was invalid. |
|
Paginated SQL results with accompanying pagination metadata. |
|
Run database queries that return paginated results. |
|
Generic pagnination cursor for keyset pagination. |
|
Holds the data returned in an RFC 8288 |
Class Inheritance Diagram¶
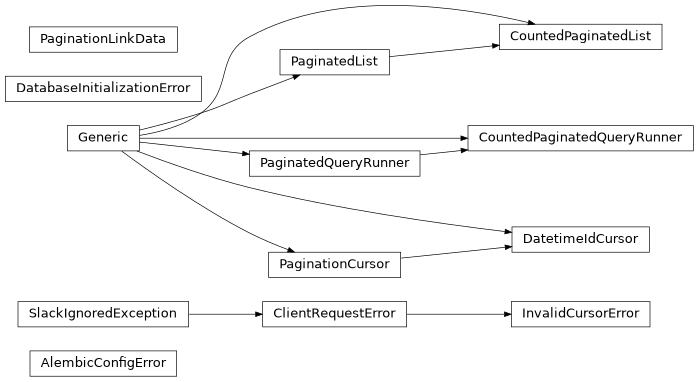
safir.datetime Package¶
Date and time manipulation utility functions.
Functions¶
|
Construct a |
|
Format a datetime for logging and human readabilty. |
|
Format a timestamp in UTC in a standard ISO date format. |
|
Parse a string in a standard ISO date format. |
|
Parse a string into a |
safir.dependencies.arq Module¶
A FastAPI dependency that supplies a Redis connection for arq.
Classes¶
A FastAPI dependency that maintains a Redis client for enqueuing tasks to the worker pool. |
Variables¶
Singleton instance of |
Class Inheritance Diagram¶
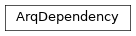
safir.dependencies.db_session Module¶
Manage an async database session.
Classes¶
Manages an async per-request SQLAlchemy session. |
Variables¶
The dependency that will return the async session proxy. |
Class Inheritance Diagram¶

safir.dependencies.gafaelfawr Module¶
Gafaelfawr authentication dependencies.
Functions¶
Retrieve Gafaelfawr delegated token from HTTP headers. |
|
|
Retrieve authentication information from HTTP headers. |
|
Logger bound to the authenticated user. |
safir.dependencies.http_client Module¶
HTTP client dependency for FastAPI.
Classes¶
Provides an |
Variables¶
Default timeout (in seconds) for outbound HTTP requests. |
|
The dependency that will return the HTTP client. |
Class Inheritance Diagram¶

safir.dependencies.logger Module¶
Logger dependency for FastAPI.
Provides a structlog
logger as a FastAPI dependency. The logger will
incorporate information from the request in its bound context.
Classes¶
Provides a structlog logger configured with request information. |
Variables¶
The dependency that will return the logger for the current request. |
Class Inheritance Diagram¶
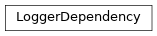
safir.dependencies.metrics Module¶
Dependencies for metrics functionality.
Classes¶
|
Provides EventManager-managed events for apps to publish. |
A blueprint for an event publisher container class. |
Class Inheritance Diagram¶
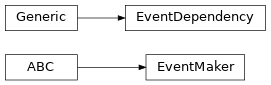
safir.fastapi Package¶
Helper code for FastAPI (other than dependencies and middleware).
Functions¶
|
Exception handler for exceptions derived from |
Classes¶
|
Represents an error in a client request. |
Class Inheritance Diagram¶

safir.gcs Package¶
Utilities for interacting with Google Cloud Storage.
Classes¶
|
Generate signed URLs for Google Cloud Storage blobs. |
Class Inheritance Diagram¶
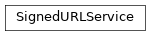
safir.github Package¶
GitHub API client factory and Pydantic models.
Classes¶
|
Factory for creating GitHub App clients authenticated either as an app or as an installation of that app. |
Class Inheritance Diagram¶

safir.github.models Module¶
Pydantic models for GitHub v3 REST API resources.
Classes¶
A Pydantic model for a blob, returned by the GitHub blob endpoint. |
|
A Pydantic model for the commit field found in |
|
A Pydantic model for a GitHub branch. |
|
|
The level of a check run output annotation. |
|
The check run conclusion state. |
A Pydantic model for the "check_run" field in a check_run webhook payload ( |
|
Check run output report. |
|
A Pydantic model of the |
|
|
The check run status. |
|
The conclusion state of a GitHub check suite. |
Brief information about a check suite in the |
|
A Pydantic model for the |
|
|
The status of a GitHub check suite. |
A Pydantic model for the last commit to the head branch of a PR. |
|
A Pydantic model for a GitHub Pull Request. |
|
|
The state of a GitHub pull request (PR). |
A Pydantic model for the |
|
A Pydantic model for the |
|
A Pydantic model for the |
Class Inheritance Diagram¶
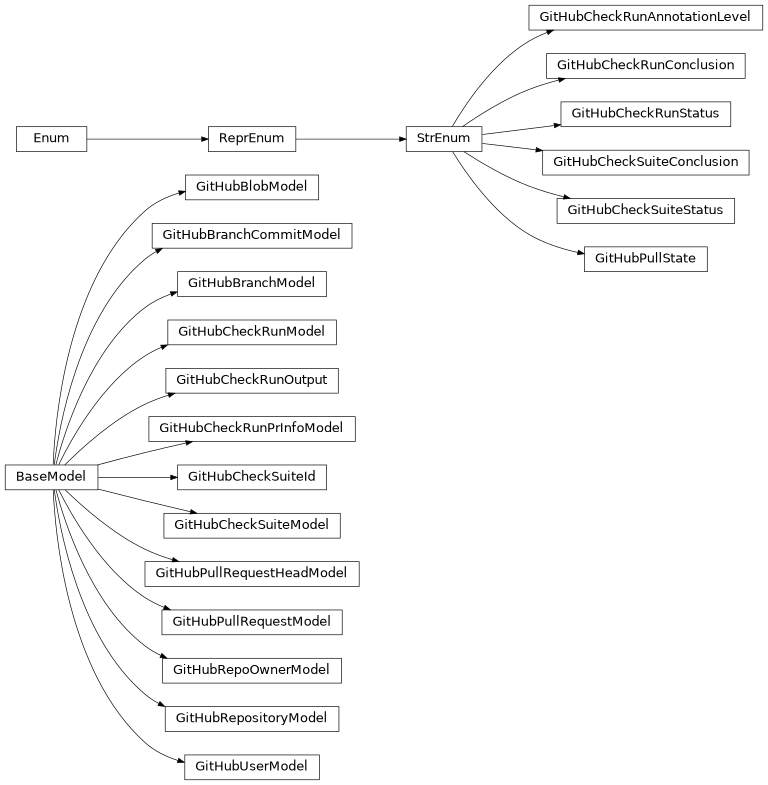
safir.github.webhooks Module¶
Pydantic models for GitHub webhook payloads.
Classes¶
|
The action performed on an GitHub App |
A Pydantic model for an |
|
A pydantic model for repository objects used by |
|
A Pydantic model for the |
|
The action performed on a GitHub App |
|
A Pydantic model for a |
|
|
The action performed in a GitHub |
A Pydantic model for the |
|
|
The action performed in a GitHub |
A Pydantic model for the |
|
|
The action performed on a GitHub |
A Pydantic model for a |
|
A Pydantic model for the |
Class Inheritance Diagram¶
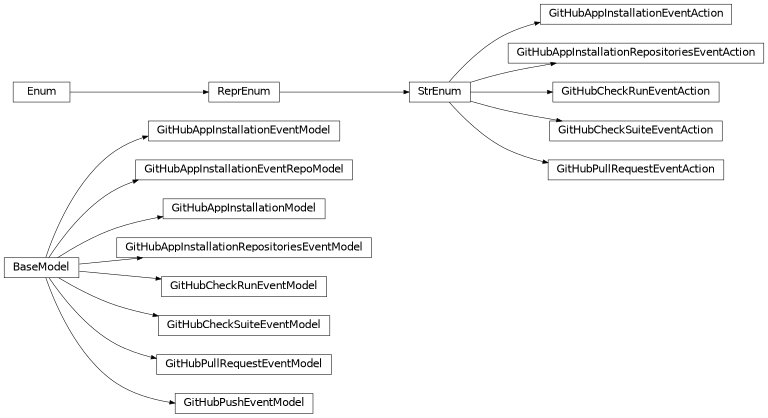
safir.kafka Package¶
Classes¶
Type for parameters to the constructor of an aiokafka client. |
|
|
Schema registry compatibility types. |
Type for parameters to the constructor of a FastStream broker. |
|
A schema is incompatible with the latest version in the registry. |
|
The decalred name or namespace for an Avro schema is not valid. |
|
The Meta inner class on a model has unexpected values in fields. |
|
Settings for connecting to Kafka. |
|
Subset of settings required for Plaintext auth. |
|
|
A manager for schemas that are represented as Pydantic models in Python, and translated into Avro for the Confluent Schema Registry. |
|
Kafka SASL mechanisms. |
Subset of settings required for SASL SSLauth. |
|
Subset of settings required for SASL PLAINTEXT auth. |
|
|
Schema and registry metadata. |
Settings for constructing a |
|
Kwargs used to construct an AsyncSchemaRegistryClient. |
|
|
Kafka SASL security protocols. |
Subset of settings required for SSL auth. |
|
The schema registry client returns None when deserializing. |
|
|
A schema is not managed by the Registry, and therefore cannot be serialized into a native Python object. |
Class Inheritance Diagram¶
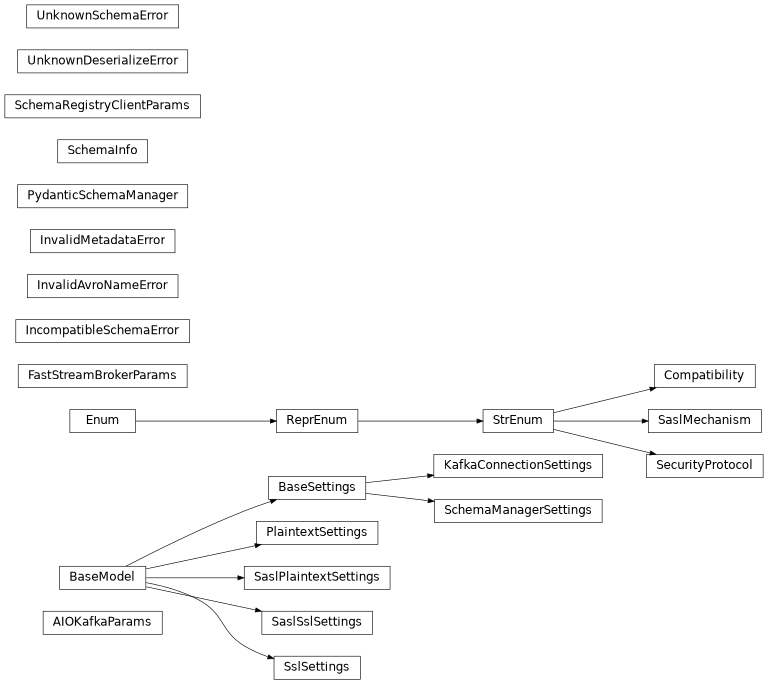
safir.kubernetes Package¶
Utilities for configuring a Kubernetes client.
Functions¶
Load the Kubernetes configuration. |
safir.logging Package¶
Utilities for configuring structlog-based logging.
Functions¶
|
Add the log level to the event dict as |
|
Set up logging for Alembic. |
|
Configure logging and structlog. |
|
Set up logging. |
Classes¶
|
Python logging level. |
|
Logging profile for the application. |
Class Inheritance Diagram¶
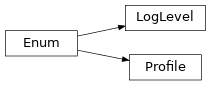
safir.metadata Package¶
Standardized metadata for Roundtable and Phalanx HTTP services.
Functions¶
|
Retrieve metadata for the application. |
|
Get a specific URL from a package's |
Classes¶
Metadata about a package. |
Class Inheritance Diagram¶

safir.metrics Package¶
Functions¶
Choose an appropriate metrics configuration based on the environment. |
Classes¶
|
Base assertion error with common attributes and messaging. |
Metrics configuration, including the required Kafka configuration. |
|
Metrics configuration when metrics reporting is disabled. |
|
|
Two publishers were registered with the same name. |
|
Interface for a client for publishing application metrics events. |
An attempt to create a publisher after manager has been initialized. |
|
Common fields for all metrics events. |
|
All event payloads should inherit from this. |
|
|
Interface for event publishers. |
Configuration for emitting events. |
|
|
A tool for publishing application metrics events. |
|
Publishes one type of event. |
Metrics configuration when enabled, including Kafka configuration. |
|
|
A topic does not exist in Kafka, or we don't have access to it. |
|
An event manager that creates mock publishers that record all publishes. |
|
Event publisher that quietly does nothing and records all payloads. |
Metrics configuration when metrics publishing is mocked. |
|
|
An event manager that creates publishers that quietly do nothing. |
|
Event publisher that quietly does nothing. |
|
Expected events were not published consecutively. |
|
Some expected items were not published. |
|
Expected has a different number of items than were published. |
|
A list of event payload models with assertion helpers. |
|
Expected more events than have actually been published. |
Variables¶
A helper object that compares equal to everything. |
|
A helper object that compares equal to everything except None. |
|
Type alias. |
Class Inheritance Diagram¶
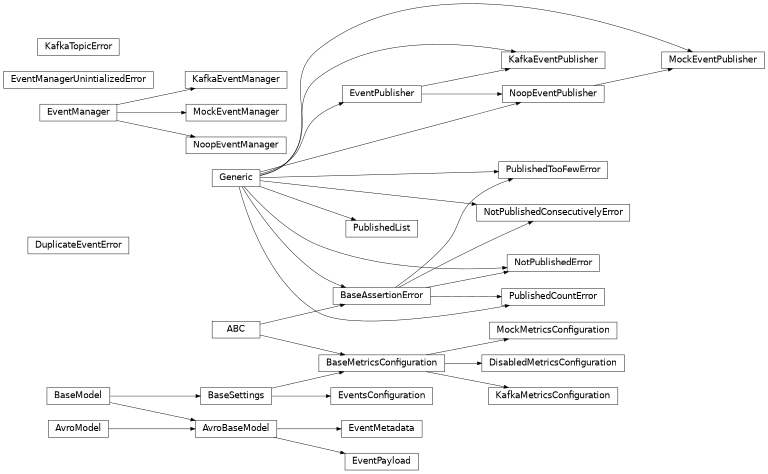
safir.models Package¶
Standard models for FastAPI applications.
Notes
FastAPI does not appear to export its error response model in a usable form, so define a copy of it so that we can reference it in API definitions to generate good documentation.
Classes¶
The detail of the error message. |
|
|
Possible locations for an error. |
A structured API error message. |
Class Inheritance Diagram¶
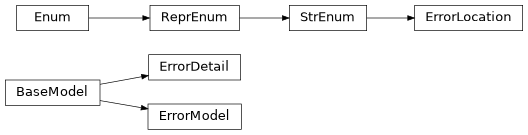
safir.middleware.ivoa Module¶
Middleware for IVOA services.
Classes¶
Make POST parameter keys all lowercase. |
|
Make query parameter keys all lowercase. |
Class Inheritance Diagram¶
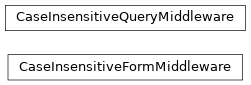
safir.middleware.x_forwarded Module¶
Update the request based on X-Forwarded-For
headers.
Classes¶
|
ASGI middleware to update the request based on |
Class Inheritance Diagram¶

safir.pydantic Package¶
Utilities for Pydantic models.
Functions¶
Pydantic field validator for datetime fields. |
|
Pydantic field validator for datetime fields in ISO format. |
|
|
Convert a string to camel case. |
|
Generate a model validator imposing a one and only one constraint. |
Classes¶
|
Class Inheritance Diagram¶

safir.redis Package¶
Redis database support.
Classes¶
|
Raised when a stored Pydantic object in Redis cannot be decoded (and possibly decrypted) or deserialized. |
|
A Pydantic-based Redis store that encrypts data. |
|
JSON-serialized encrypted storage in Redis. |
Class Inheritance Diagram¶

safir.sentry Package¶
Sentry helpers.
Functions¶
|
Add the env to the fingerprint, and enrich from |
|
Return the time spent in a span (to the present if not finished). |
|
Add the environment to the event fingerprint. |
|
Add tags and context from |
Classes¶
|
Enriches the Sentry context when paired with the |
|
Parent class of exceptions arising from HTTPX failures. |
Class Inheritance Diagram¶

safir.slack.blockkit Module¶
Slack Block Kit message models.
Classes¶
Base class for any Slack Block Kit block. |
|
Base class for Slack Block Kit blocks for the |
|
A component of a Slack message with a heading and a code block. |
|
An attachment in a Slack message with a heading and text body. |
|
|
Parent class of exceptions that can be reported to Slack. |
Message to post to Slack. |
|
A component of a Slack message with a heading and a text body. |
|
One field in a Slack message with a heading and text body. |
|
|
Parent class of exceptions arising from HTTPX failures. |
Class Inheritance Diagram¶
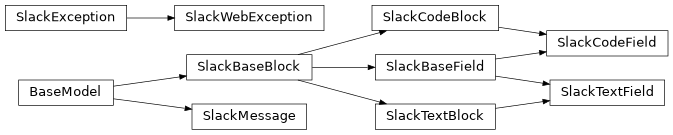
safir.slack.webhook Module¶
Send messages to Slack.
Classes¶
Parent class for exceptions that should not be reported to Slack. |
|
|
Custom |
|
Send messages to a Slack webhook. |
Class Inheritance Diagram¶
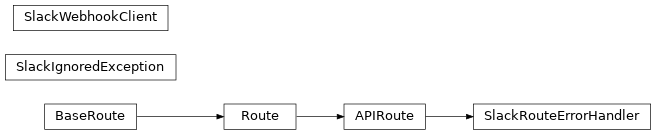
safir.testing.containers Package¶
Safir-provided test containers.
Classes¶
|
Kafka container. |
|
A Testcontainers Confluent schema registry container. |
Class Inheritance Diagram¶
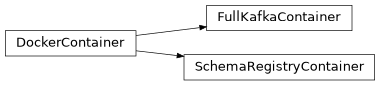
safir.testing.gcs Package¶
Mock Google Cloud Storage API for testing.
Functions¶
|
Replace the Google Cloud Storage API with a mock class. |
Classes¶
|
Mock version of |
|
Mock version of |
|
Mock version of |
Class Inheritance Diagram¶
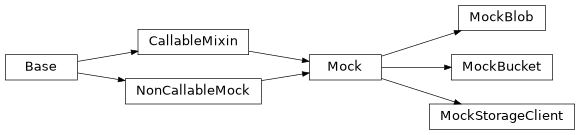
safir.testing.kubernetes Package¶
Mock Kubernetes API and utility functions for testing.
Functions¶
Replace the Kubernetes API with a mock class. |
|
|
Strip |
Classes¶
Mock Kubernetes API for testing. |
Class Inheritance Diagram¶
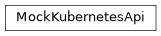
safir.testing.logging Package¶
Helper functions for testing logging.
Functions¶
|
Parse JSON log record tuples into structured data. |
safir.testing.sentry Package¶
Helpers for testing Sentry reporting.
Functions¶
|
Return a function that returns a container with items sent to Sentry. |
Return an init function that injects a no-op transport. |
Classes¶
|
Contents and metadata of a Sentry attachment. |
|
A container for interesting items sent to Sentry. |
A transport that doesn't actually transport anything. |
Class Inheritance Diagram¶
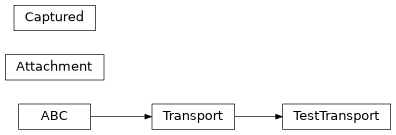
safir.testing.slack Package¶
Mock Slack server for testing Slack messaging.
Functions¶
|
Set up a mocked Slack server. |
Classes¶
|
Represents a Slack incoming webhook and remembers what was posted. |
Class Inheritance Diagram¶
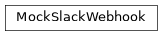
safir.testing.uvicorn Package¶
Utility functions for managing an external Uvicorn test process.
Functions¶
|
Spawn an ASGI app as a separate Uvicorn process. |
Classes¶
Timeout waiting for the server to start listening. |
|
|
Properties of the running Uvicorn service. |
Class Inheritance Diagram¶
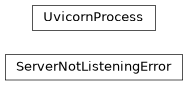
safir.testing.uws Package¶
Mocks and functions for testing services using the Safir UWS support.
Functions¶
|
Assert that two job XML documents are equal. |
|
Set up the mock for a Wobbly server. |
Classes¶
|
Simulate execution of jobs with a mock queue. |
Mock the Wobbly web service, which stores UWS job information. |
Class Inheritance Diagram¶
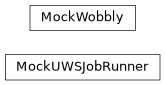
safir.uws Package¶
Support library for writing UWS-enabled services.
Classes¶
A single UWS job with deserialized parameters. |
|
Fields common to all variations of the job record. |
|
Information required to create a new UWS job (Wobbly format). |
|
Failure information about a job. |
|
A single result from a job. |
|
Input model when aborting a job. |
|
Input model when marking a job as complete. |
|
Input model when marking a job as failed. |
|
Input model when marking a job as executing. |
|
Input model when updating job metadata. |
|
Input model when marking a job as queued. |
|
|
Unsupported value passed to a parameter. |
Defines the interface for a model suitable for job parameters. |
|
A single UWS job (Wobbly format). |
|
Settings common to all applications using the UWS library. |
|
|
Glue between a FastAPI application and the UWS implementation. |
|
Configuration for the UWS service. |
|
An error with an associated error code. |
|
Defines a FastAPI dependency to get the UWS job parameters. |
|
Invalid parameters were passed to a UWS API. |
Class Inheritance Diagram¶
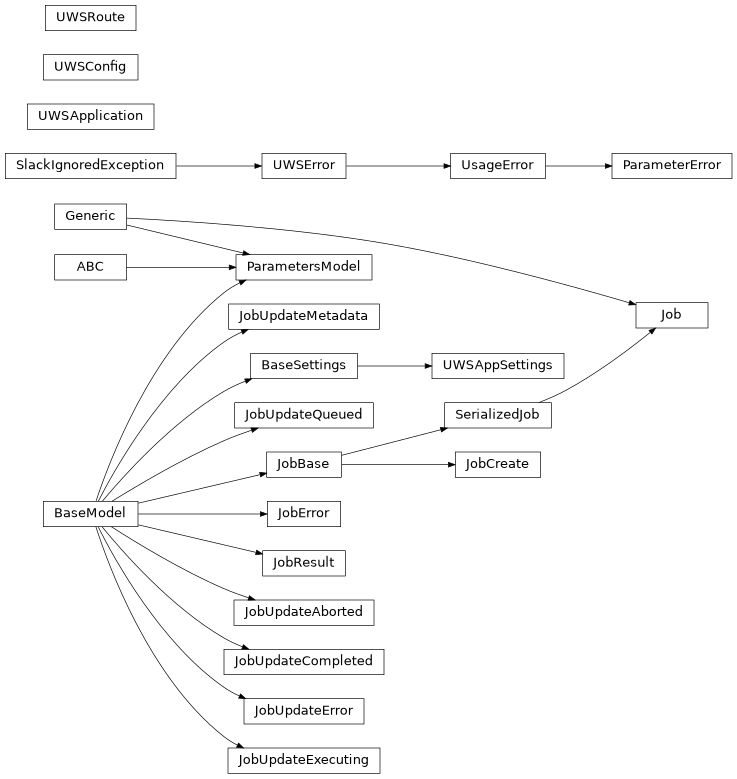