API reference#
safir Package#
Safir is the Rubin Observatory’s library for building FastAPI services for the Rubin Science Platform.
Variables#
The version string of Safir (PEP 440 / SemVer compatible). |
|
The decomposed version, split across " |
safir.arq Module#
An arq client with a mock for testing.
Classes#
|
A base class for errors related to arq jobs. |
|
The job was not successfully queued. |
|
A job cannot be found. |
|
The job's result is unavailable. |
|
Mode configuration for the Arq queue. |
|
Information about a queued job. |
|
The full result of a job, as well as its metadata. |
|
An common interface for working with an arq queue that can be implemented either with a real Redis backend, or an in-memory repository for testing. |
|
A distributed queue, based on arq and Redis. |
|
A mocked queue for testing API services. |
Class Inheritance Diagram#
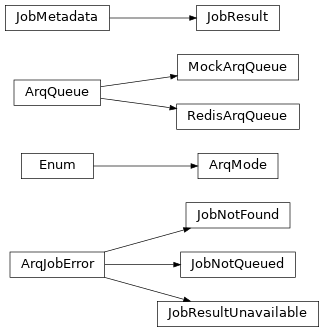
safir.asyncio Module#
Utility functions for asyncio code.
Functions#
Run the decorated function with asyncio.run. |
safir.database Module#
Utility functions for database management.
Functions#
|
Create a new async database session. |
|
Create a new async database engine. |
|
Create a new sync database session. |
|
Add the UTC time zone to a naive datetime from the database. |
|
Strip time zone for storing a datetime in the database. |
|
Create and initialize a new database. |
Classes#
Database initialization failed. |
Class Inheritance Diagram#

safir.datetime Module#
Date and time manipulation utility functions.
Functions#
Construct a ~datetime.datetime for the current time. |
|
|
Format a timestamp in UTC in a standard ISO date format. |
|
Parse a string in a standard ISO date format. |
safir.dependencies.arq Module#
A FastAPI dependency that supplies a Redis connection for arq.
Classes#
A FastAPI dependency that maintains a Redis client for enqueing tasks to the worker pool. |
Variables#
Singleton instance of ArqDependency that serves as a FastAPI dependency. |
Class Inheritance Diagram#
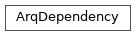
safir.dependencies.db_session Module#
Manage an async database session.
Classes#
Manages an async per-request SQLAlchemy session. |
Variables#
The dependency that will return the async session proxy. |
Class Inheritance Diagram#

safir.dependencies.gafaelfawr Module#
Gafaelfawr authentication dependencies.
Functions#
|
Retrieve authentication information from HTTP headers. |
|
Logger bound to the authenticated user. |
safir.dependencies.http_client Module#
HTTP client dependency for FastAPI.
Classes#
Provides an |
Variables#
Default timeout (in seconds) for outbound HTTP requests. |
|
The dependency that will return the HTTP client. |
Class Inheritance Diagram#

safir.dependencies.logger Module#
Logger dependency for FastAPI.
Provides a structlog logger as a FastAPI dependency. The logger will incorporate information from the request in its bound context.
Classes#
Provides a structlog logger configured with request information. |
Variables#
The dependency that will return the logger for the current request. |
Class Inheritance Diagram#
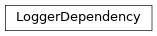
safir.gcs Module#
Utilities for interacting with Google Cloud Storage.
Classes#
|
Generate signed URLs for Google Cloud Storage blobs. |
Class Inheritance Diagram#
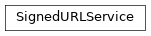
safir.kubernetes Module#
Utilities for configuring a Kubernetes client.
Functions#
Load the Kubernetes configuration. |
Variables#
safir.logging Module#
Utilities for configuring structlog-based logging.
Functions#
|
Add the log level to the event dict as |
|
Configure logging and structlog. |
|
Set up logging. |
Classes#
|
Python logging level. |
|
Logging profile for the application. |
Variables#
Name of the configured global logger. |
Class Inheritance Diagram#
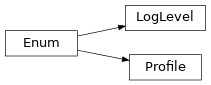
safir.metadata Module#
Standardized metadata for Roundtable HTTP services.
Functions#
|
Retrieve metadata for the application. |
|
Get a specific URL from a package's |
Classes#
|
Metadata about a package. |
Class Inheritance Diagram#

safir.models Module#
Standard models for FastAPI applications.
Notes
FastAPI does not appear to export its error response model in a usable form, so define a copy of it so that we can reference it in API definitions to generate good documentation.
Classes#
|
The detail of the error message. |
|
Possible locations for an error. |
|
A structured API error message. |
Class Inheritance Diagram#
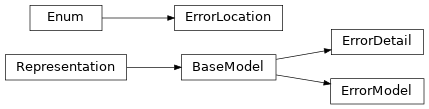
safir.middleware.ivoa Module#
Middleware for IVOA services.
Classes#
|
Make query parameter keys all lowercase. |
Class Inheritance Diagram#

safir.middleware.x_forwarded Module#
Update the request based on X-Forwarded-For
headers.
Classes#
|
Middleware to update the request based on |
Class Inheritance Diagram#

safir.pydantic Module#
Utility functions for Pydantic models.
Functions#
Pydantic validator for datetime fields. |
|
Pydantic validator for datetime fields in ISO format. |
|
|
Convert a string to camel case. |
|
Generate a validator imposing a one and only one constraint. |
Classes#
|
pydantic.BaseModel configured to accept camel-case input. |
Class Inheritance Diagram#

safir.testing.gcs Module#
Mock Google Cloud Storage API for testing.
Functions#
|
Replace the Google Cloud Storage API with a mock class. |
Classes#
|
Mock version of |
|
Mock version of |
|
Mock version of |
Class Inheritance Diagram#
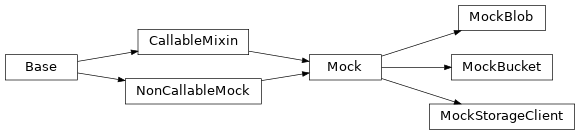
safir.testing.kubernetes Module#
Mock Kubernetes API for testing.
Functions#
Replace the Kubernetes API with a mock class. |
Classes#
Mock Kubernetes API for testing. |
Class Inheritance Diagram#
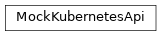